[ad_1]
GitHub Motion integration with Playwright allows seamless automated testing and deployment workflows for internet functions. GitHub Actions, the platform’s automation software, permits these exams to be triggered routinely upon code modifications, making certain fast suggestions and environment friendly bug detection.
This integration empowers groups to construct, take a look at, and deploy with confidence, automating repetitive duties and enhancing general growth productiveness. By combining the flexibility of Playwright with the automation capabilities of GitHub Actions, builders can streamline their workflows, delivering high-quality internet functions with velocity and precision.
What Is Playwright?
Microsoft Playwright is an open-source automation framework for end-to-end testing, browser automation, and internet scraping. Developed by Microsoft, Playwright gives a unified API to automate interactions with internet browsers like Microsoft Edge, Google Chrome, and Mozilla Firefox. It permits builders to put in writing scripts in numerous programming languages, together with Java, Python, JavaScript, and C#.
Listed below are some key options of Playwright:
- Multi-Browser Assist: Playwright helps a number of internet browsers, together with Firefox, Chrome, Safari, and Microsoft Edge. This enables builders and testers to run their exams on totally different browsers with a constant API.
- Headless and Headful Modes: Playwright can run browsers in each headless mode (with out a graphical interface) and headful mode (with a graphical interface), offering flexibility for various use instances.
- Cross-Browser Testing: Playwright permits you to write exams that run on a number of browsers and platforms, making certain your internet utility works accurately throughout totally different platforms.
- Emulation of Cell Gadgets and Contact Occasions: Playwright can emulate numerous cellular gadgets and simulate contact occasions, enabling you to check how your internet utility behaves on totally different cellular gadgets.
- Parallel Check Execution: Playwright helps parallel take a look at execution, permitting you to run exams concurrently, decreasing the general take a look at suite execution time.
- Seize Screenshots and Movies: Playwright can seize screenshots and file movies throughout take a look at execution, serving to you visualize the habits of your utility throughout exams.
- Intercept Community Requests: You may intercept and modify community requests and responses, which is helpful for testing situations involving AJAX requests and APIs.
- Auto-Ready for Parts: Playwright routinely waits for components to be prepared earlier than performing actions, decreasing the necessity for guide waits and making exams extra dependable.
- Web page and Browser Contexts: Playwright permits you to create a number of browser contexts and pages, enabling environment friendly administration of browser cases and remoted environments for testing.
What Is GitHub Actions?
GitHub Actions is an automation platform provided by GitHub that streamlines software program growth workflows. It empowers customers to automate a wide selection of duties inside their growth processes. By leveraging GitHub Actions, builders/qa engineers can craft personalized workflows which might be initiated by particular occasions corresponding to code pushes, pull requests, or difficulty creation. These workflows can automate important duties like constructing functions, operating exams, and deploying code.
Primarily, GitHub Actions gives a seamless approach to automate numerous features of the software program growth lifecycle straight out of your GitHub repository.
How GitHub Actions Efficient in Automation Testing
GitHub Actions is a strong software for automating numerous workflows, together with QA automation testing. It permits you to automate your software program growth processes straight inside your GitHub repository. Listed below are some methods GitHub Actions might be efficient in QA automation testing:
1. Steady Integration (CI)
GitHub Actions can be utilized for steady integration, the place automated exams are triggered each time there’s a new code commit or a pull request. This ensures that new code modifications don’t break present performance. Automated exams can embody unit exams, integration exams, and end-to-end exams.
2. Numerous Check Environments
GitHub Actions helps operating workflows on totally different working techniques and environments. That is particularly helpful for QA testing, because it permits you to take a look at your utility on numerous platforms and configurations to make sure compatibility and establish platform-specific points.
3. Parallel Check Execution
GitHub Actions permits you to run exams in parallel, considerably decreasing the time required for take a look at execution. Parallel testing is crucial for giant take a look at suites, because it helps in acquiring sooner suggestions on the code modifications.
4. Customized Workflows
You may create customized workflows tailor-made to your QA automation wants. For instance, you’ll be able to create workflows that run particular exams primarily based on the recordsdata modified in a pull request. This focused testing method helps in validating particular modifications and reduces the general testing time.
5. Integration With Testing Frameworks
GitHub Actions can seamlessly combine with well-liked testing frameworks and instruments. Whether or not you’re utilizing Selenium, Cypress, Playwright for internet automation, Appium for cellular automation, or another testing framework, you’ll be able to configure GitHub Actions to run your exams utilizing these instruments
Within the subsequent part, you will notice how we are able to combine GitHub Actions with Playwright to execute the take a look at instances.
Set Up CI/CD GitHub Actions to Run Playwright Checks
Pre-Situation
The consumer ought to have a GitHub account and already be logged in.
Use Circumstances
For automation functions, we’re taking two examples, certainly one of UI and the opposite of API.
Instance 1
Beneath is an instance of a UI take a look at case the place we log in to the location https://talent500.co/auth/signin. After a profitable login, we log off from the applying.
// @ts-check
const { take a look at, anticipate } = require("@playwright/take a look at");
take a look at.describe("UI Check Case with Playwright", () => {
take a look at("UI Check Case", async ({ web page }) => {
await web page.goto("https://talent500.co/auth/signin");
await web page.locator('[name="email"]').click on();
await web page.locator('[name="email"]').fill("applitoolsautomation@yopmail.com");
await web page.locator('[name="password"]').fill("Check@123");
await web page.locator('[type="submit"]').nth(1).click on();
await web page.locator('[alt="DropDown Button"]').click on();
await web page.locator('[data-id="nav-dropdown-logout"]').click on();
});
});
Instance 2
Beneath is an instance of API testing, the place we automate utilizing the endpoint https://reqres.in/api for a GET request.
Confirm the next:
- GET request with Legitimate 200 Response
- GET request with InValid 404 Response
- Verification of consumer particulars
// @ts-check
const { take a look at, anticipate } = require("@playwright/take a look at");
take a look at.describe("API Testing with Playwright", () => {
const baseurl = "https://reqres.in/api";
take a look at("GET API Request with - Legitimate 200 Response", async ({ request }) => {
const response = await request.get(`${baseurl}/customers/2`);
anticipate(response.standing()).toBe(200);
});
take a look at("GET API Request with - Invalid 404 Response", async ({ request }) => {
const response = await request.get(`${baseurl}/usres/invalid-data`);
anticipate(response.standing()).toBe(404);
});
take a look at("GET Request - Confirm Person Particulars", async ({ request }) => {
const response = await request.get(`${baseurl}/customers/2`);
const responseBody = JSON.parse(await response.textual content());
anticipate(response.standing()).toBe(200);
anticipate(responseBody.knowledge.id).toBe(2);
anticipate(responseBody.knowledge.first_name).toBe("Janet");
anticipate(responseBody.knowledge.last_name).toBe("Weaver");
anticipate(responseBody.knowledge.e mail).toBeTruthy();
});
});
Steps For Configuring GitHub Actions
Step 1: Create a New Repository
Create a repository. On this case, let’s identify it “Playwright_GitHubAction.”
Step 2: Set up Playwright
Set up Playwright utilizing the next command:
npm init playwright@newest
Or
Step 3: Create Workflow
Outline your workflow within the YAML file. Right here’s an instance of a GitHub Actions workflow that’s used to run Playwright take a look at instances.
On this instance, the workflow is triggered on each push and pull request. It units up Node.js, installs undertaking dependencies, after which runs npx playwright take a look at
to execute Playwright exams.
Add the next .yml
file beneath the trail .github/workflows/e2e-playwright.yml
in your undertaking.
identify: GitHub Motion Playwright Checks
on:
push:
branches: [main]
pull_request:
branches: [main]
jobs:
take a look at:
timeout-minutes: 60
runs-on: ubuntu-latest
steps:
- makes use of: actions/checkout@v3
- makes use of: actions/setup-node@v3
with:
node-version: 18
- identify: Set up dependencies
run: npm ci
- identify: Set up Playwright Browsers
run: npx playwright set up --with-deps
- identify: Run Playwright exams
run: npx playwright take a look at
- makes use of: actions/upload-artifact@v3
if: all the time()
with:
identify: playwright-report
path: playwright-report/
retention-days: 10
Right here’s a breakdown of what this workflow does:
Set off Situations
The workflow is triggered on push occasions to the primary
department.
Job Configuration
- Job identify: e2e-test
- Timeout: 60 minutes (the job will terminate if it runs for greater than 60 minutes)
- Working system: ubuntu-latest
Steps
- Try the repository code utilizing
actions/checkout@v3
. - Arrange Node.js model 18 utilizing
actions/setup-node@v3
. - Set up undertaking dependencies utilizing
npm ci
. - Set up Playwright browsers and their dependencies utilizing
npx playwright set up --with-deps
. - Run Playwright exams utilizing
npx playwright take a look at
. - Add the take a look at report listing (
playwright-report/
) as an artifact utilizingactions/upload-artifact@v3
. This step all the time executes (if: all the time()
), and the artifact is retained for 10 days.
Check outcomes might be saved within the playwright-report/
listing.
Beneath is the folder construction the place you’ll be able to see the .yml
file and take a look at instances beneath the exams folder to execute.
Execute the Check Circumstances
Commit your workflow file (e2e-playwright.yml
) and your Playwright take a look at recordsdata. Push the modifications to your GitHub repository. GitHub Actions will routinely choose up the modifications and run the outlined workflow.
As we push the code, the workflow begins to run routinely.
Click on on the above hyperlink to open the e2e-test.
Click on on e2e-test. Within the display screen under, you’ll be able to see the code being checked out from GitHub, and the browsers begin putting in.
As soon as all dependencies and browsers are put in, the take a look at instances begin executing. Within the screenshot under, you’ll be able to see all 12 take a look at instances handed in three browsers (Firefox, Chrome, and WebKit).
HTML Report
Click on on the playwright-report hyperlink from the Artifacts part. An HTML report is generated regionally
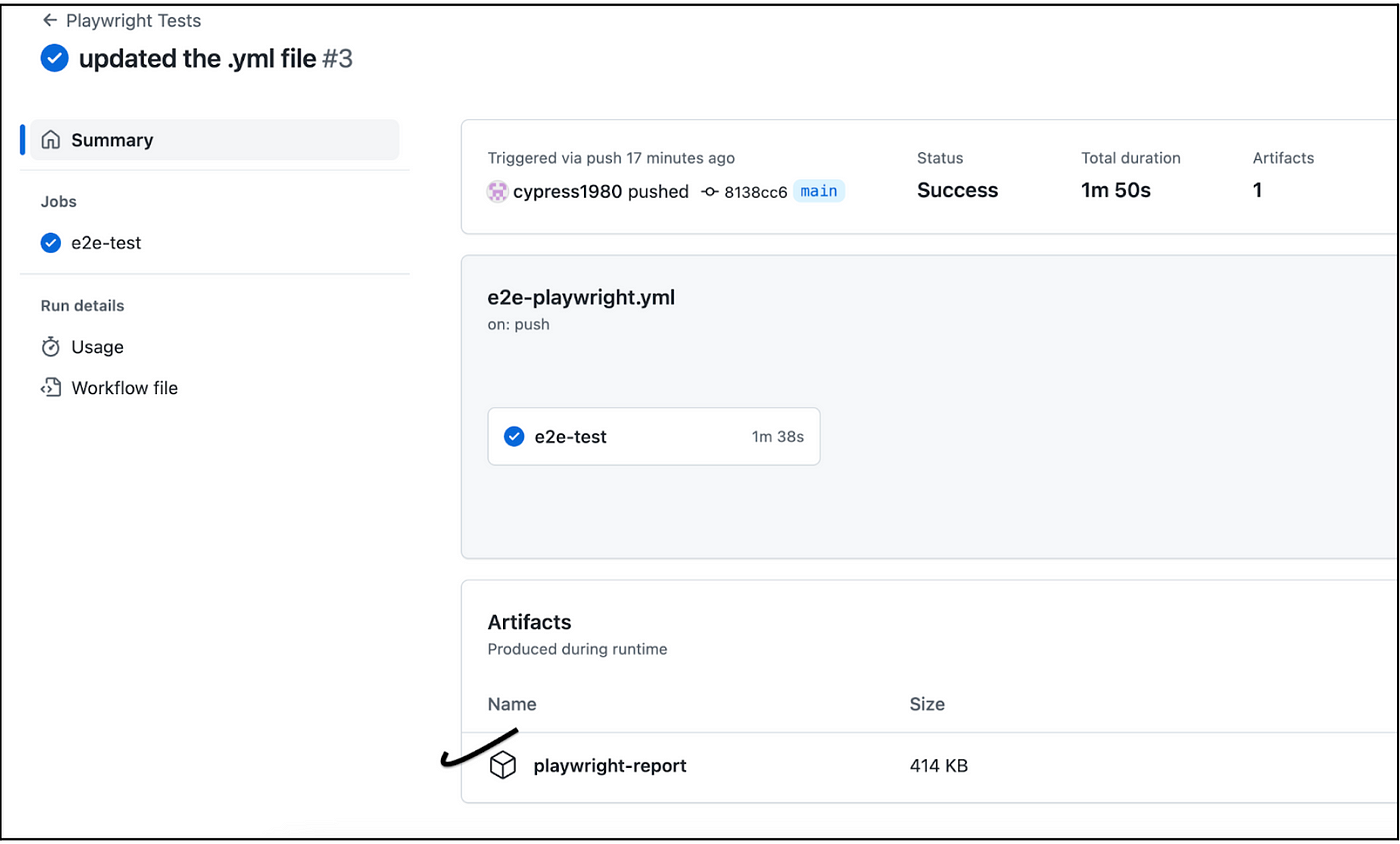
Click on the hyperlink above to view the HTML report. Each API and UI take a look at instances are handed.
Wrapping Up
GitHub Actions automate the testing course of, making certain each code change is totally examined with out guide intervention. Playwright’s capacity to check throughout numerous browsers and platforms ensures a complete analysis of your utility’s performance.
By combining GitHub Actions and Playwright, builders can streamline workflows, guarantee code high quality, and in the end ship higher consumer experiences.
[ad_2]